I wanted to experiment with making an actuator that was flat but still had high torque. I haven't gotten around to making one yet but I have experimented with magnets to see what the fields look like. I'm not sure what all I can do with this so far but here's the code:
/*[Ball]*/
Show_ball = true;
Ball_size = 7; //[1:.01:100]
Ball_verticle_spacing = 20; //[1:.1:200]
Ball_horizontal_spacing = 51.42; //[1:.01:200]
Move_ball_horizontal = 20; //[0:.01:100]
Move_ball_X = 0; //[-200:.1:200]
Move_ball_Y = 0; //[-200:.1:200]
Move_ball_Z = 0; //[-200:.1:200]
/*[Groove]*/
Show_Groove = true;
/*[Outer_Race]*/
Show_race1 = true;
Race1_spacing = 0; //[0:.01:20]
Race1_inside_diameter = 42.5; //[0:.1:200]
Race1_outside_diameter= 65; //[0:.1:200]
Race1_height = 0 ; //[-20:.01:100]
Race1_inside_shape = 200; //[0:1:200]
Race1_outside_shape = 200; //[0:1:200]
Rotate_Race1_X = 0; //[0:.1:360]
Rotate_Race1_Y= 0; //[0:.1:360]
Rotate_Race1_Z = 0; //[0:.1:360]
Move_race1_X = 0; //[0:.1:200]
Move_race1_Y = 0; //[0:.1:200]
Move_race1_Z = 0; //[0:.1:200]
/*[Inner_Race]*/
Show_race2 = true;
Race2_spacing = 0; //[0:.01:20]
Race2_inside_diameter = 12; //[0:.1:200]
Race2_outside_diameter= 38; //[0:.1:200]
Race2_height = 0 ; //[-20:.01:100]
Race2_inside_shape = 200; //[0:1:200]
Race2_outside_shape = 200; //[0:1:200]
Rotate_Race2_X = 0; //[0:.1:360]
Rotate_Race2_Y= 0; //[0:.1:360]
Rotate_Race2_Z = 0; //[0:.1:360]
Move_race2_X = 0; //[0:.1:200]
Move_race2_Y = 0; //[0:.1:200]
Move_race2_Z = 0; //[0:.1:200]
/*[Cage]*/
Show_Cage = true;
Cage_outside_diameter = 41; //[0:.01:200]
Cage_inside_diameter = 39.5; //[.1:.01:200]
Cage_height = 0 ; //[-20:.01:100]
Ball_clearance = 0.2; //[0:.1:100]
Inside_shape = 200; //[3:1:200]
Outside_shape = 200; //[3:1:200]
Rotate_Cage_X = 0; //[0:.1:360]
Rotate_Cage_Y = 0; //[0:.1:360]
Rotate_Cage_Z = 0; //[0:.1:360]
Move_cage_X = 0; //[0:.1:200]
Move_cage_Y = 0; //[0:.1:200]
Move_cage_Z = 0; //[0:.1:200]
/*[Rows and Columns]*/
Columns = 1; //[1:20]
Rows = 7; //[1:360]
/*[Taper]*/
Taper = 1; //[1:1:20]
/*[Rotate]*/
Rotate_All_X = 0; //[0:.1:360]
Rotate_All_Y = 0; //[0:.1:360]
Rotate_All_Z = 0; //[0:.1:360]
/*[Outer Magnet Holes]*/
Show_outer_magnets = true;
Hole_size = 7; //[1:.01:100]
Hole_depth = -1; //[-1:.01:100]
Hole_height= 25; // [.1:.01:100]
Hole_Ring_diameter = 28; //[0:.01:100]
Hole_number = 7; //[1:360]
Hole_shape = 4; //[3:1:100]
Hole_rotate = 45; //[30:.01:120]
/*[Inner Magnet Holes]*/
Show_inner_magnets = true;
Im_Hole_size = 7; //[1:.01:100]
Im_Hole_depth = -1; //[-1:.01:100]
Im_Hole_height= 25; // [.1:.01:100]
Im_Hole_Ring_diameter = 12; //[0:.01:100]
Im_Hole_number = 7; //[1:360]
Im_Hole_shape = 4; //[3:1:100]
Im_Hole_rotate = 45; //[30:.01:120]
module Ball(){
for(v=[1:Columns]){
rotate([Rotate_All_X,Rotate_All_Y,Rotate_All_Z])
translate([Move_ball_X,Move_ball_Y,Move_ball_Z-9])
translate([0,0,v*Ball_verticle_spacing-.5])
for(h=[1:Rows])
rotate([0,0,h*Ball_horizontal_spacing])
translate([0,-Move_ball_horizontal,0])
sphere(Ball_size/2,$fn=100);
}}
module BallClearance(){
for(v=[1:Columns]){
rotate([Rotate_All_X,Rotate_All_Y,Rotate_All_Z])
translate([Move_ball_X,Move_ball_Y,Move_ball_Z-9])
translate([0,0,v*Ball_verticle_spacing-.5])
for(h=[1:Rows])
rotate([0,0,h*Ball_horizontal_spacing])
translate([0,-Move_ball_horizontal,0])
sphere(Ball_size/2+ Ball_clearance,$fn=100);
}}
module groove(){
if(Show_Groove)
for(g=[1:Columns]){
rotate([Rotate_All_X,Rotate_All_Y,Rotate_All_Z])
translate([Move_ball_X,Move_ball_Y,Move_ball_Z-9])
translate([0,0,g*Ball_verticle_spacing-.5])
rotate_extrude(convexity = 10,$fn=100)
translate([Cage_outside_diameter/2, 0, 0])
circle(r = Ball_size/2+.5);
}}
module Cage(){
if (Show_Cage)
translate([Move_cage_X,Move_cage_Y,Move_cage_Z])
rotate([Rotate_All_X,Rotate_All_Y,Rotate_All_Z])
rotate([Rotate_Cage_X,Rotate_Cage_Y,Rotate_Cage_Z])
linear_extrude(Ball_verticle_spacing*Columns+Cage_height,scale=Taper,convexity=11)
difference(){
circle(Cage_outside_diameter/2,$fn=Outside_shape);
circle(Cage_inside_diameter/2,$fn=Inside_shape);
}}
module Race1(){
if (Show_race1)
translate([Move_race1_X,Move_race1_Y,Move_race1_Z])
rotate([Rotate_All_X,Rotate_All_Y,Rotate_All_Z])
rotate([Rotate_Race1_X,Rotate_Race1_Y,Rotate_Race1_Z])
linear_extrude(Ball_verticle_spacing*Columns+Race1_height,scale=Taper,convexity=11)
difference(){
circle(Race1_outside_diameter/2+Race1_spacing,$fn=Race1_outside_shape);
circle(Race1_inside_diameter/2+Race1_spacing,$fn=Race1_inside_shape);
}}
module Race2(){
if (Show_race2)
translate([Move_race2_X,Move_race2_Y,Move_race2_Z])
rotate([Rotate_All_X,Rotate_All_Y,Rotate_All_Z])
rotate([Rotate_Race2_X,Rotate_Race2_Y,Rotate_Race2_Z])
linear_extrude(Ball_verticle_spacing*Columns+Race2_height,scale=Taper,convexity=11)
difference(){
circle(Race2_outside_diameter/2+Race2_spacing,$fn=Race2_outside_shape);
circle(Race2_inside_diameter/2+Race2_spacing,$fn=Race2_inside_shape);
}}
module OuterMagnetHoles(){
if (Show_outer_magnets)
rotate([Rotate_All_X,Rotate_All_Y,Rotate_All_Z])
translate([0,0,Hole_depth])
linear_extrude(Hole_height+2,convexity=11)
for(h=[1:Hole_number])
rotate([0,0,h*360/Hole_number])
translate([0,-Hole_Ring_diameter,0])
rotate([0,0,Hole_rotate])
circle(Hole_size/2,$fn=Hole_shape);
}
module InnerMagnetHoles(){
if(Show_inner_magnets)
rotate([Rotate_All_X,Rotate_All_Y,Rotate_All_Z])
translate([0,0,Im_Hole_depth])
linear_extrude(Im_Hole_height+2,convexity=11)
for(h=[1:Im_Hole_number])
rotate([0,0,h*360/Im_Hole_number])
translate([0,-Im_Hole_Ring_diameter,0])
rotate([0,0,Im_Hole_rotate])
circle(Im_Hole_size/2,$fn=Im_Hole_shape);
}
module Show_ball(){
if (Show_ball)
Ball();
}
difference(){
Race1();
Ball();
groove();
OuterMagnetHoles();
}
difference(){
Race2();
Ball();
groove();
InnerMagnetHoles();
}
difference(){
Cage();
Ball();
BallClearance();
}
Show_ball();
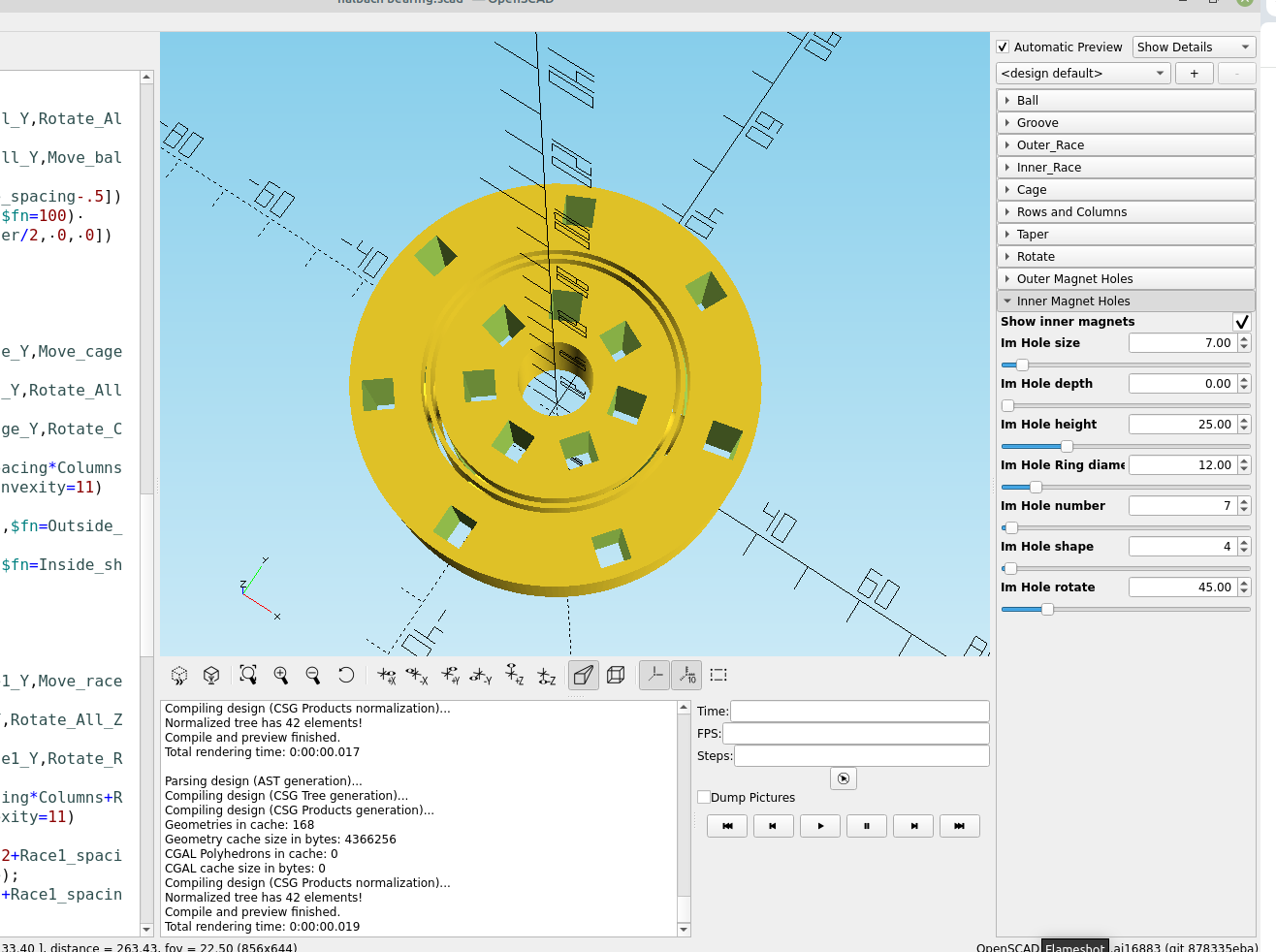
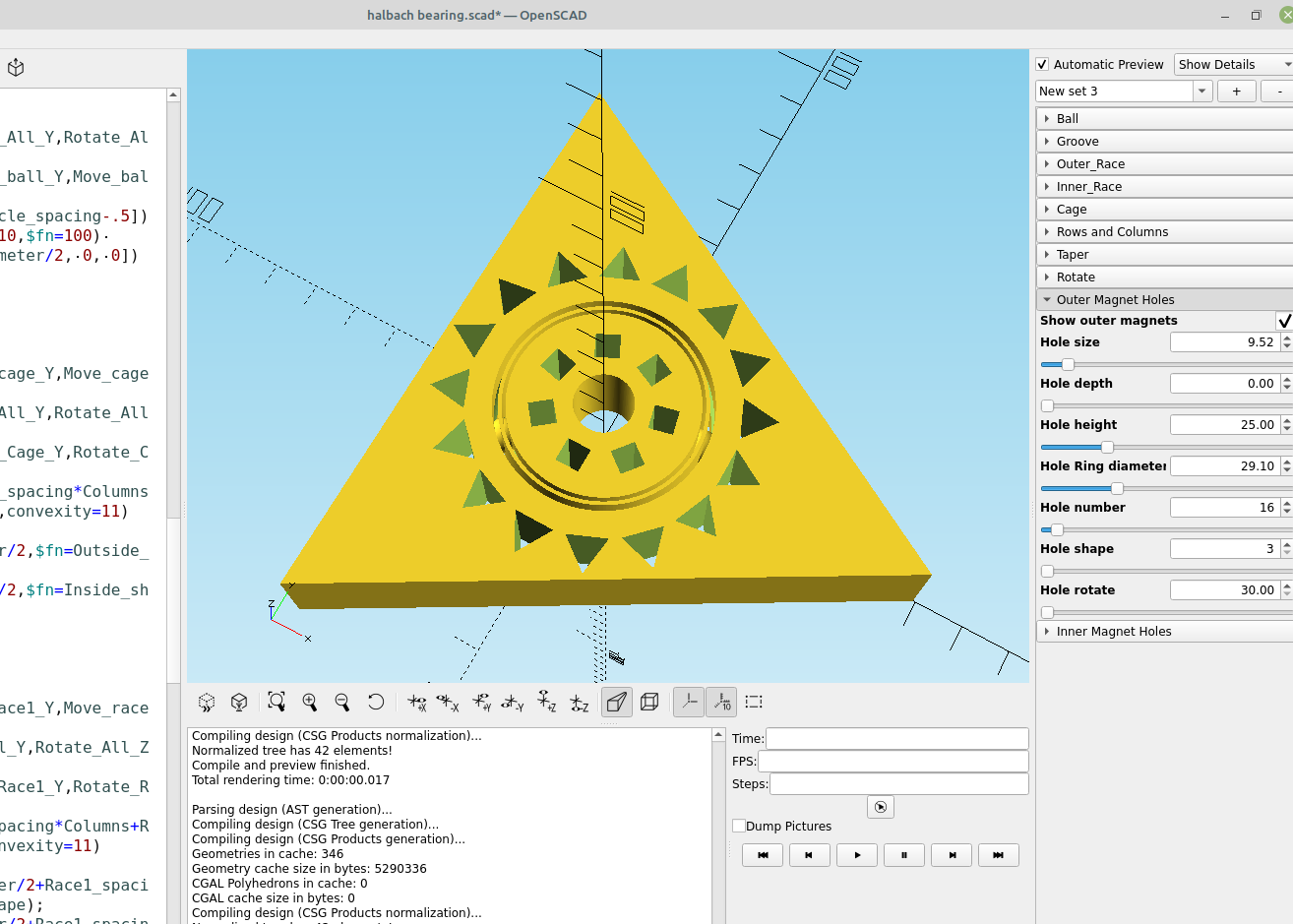