When I was making a frame for a round lithophane I needed to make some circular text, there are several good examples on the internet but I wanted something that was more customizable.
Here is the code:
/*[Circle Text 1]*/
text1 = "123";
Font1 = "";
size1=6;//[1:.01:20]
height1=2;//[1:.01:10]
spacing1=20;//[5:.01:100]
rotation1=12;//[0:.01:180]
diameter1=23;//[1.01:200]
rotate_x=0;//[0:.01:360]
rotate_y=0;//[0:.01:360]
rotate_z=0;//[0:.01:360]
length1 = len(text1);
/*[Circle Text 2]*/
text2 = "456";
Font2 = "";
size2=6;//[1:.01:20]
text_height2=2;//[1:.01:10]
rotation2=134;//[0:.01:180]
spacing2=20;//[5:.01:100]
diameter2=16.5;//[1.01:200]
rotate_x2=0;//[0:.01:360]
rotate_y2=0;//[0:.01:360]
rotate_z2=0;//[0:.01:360]
length2 = len(text2);
module CircleText1() {
for(a=[0:length1]) {
rotate([0,length1/2*rotation1-(a*spacing1),270])
translate([diameter1,0,0])
rotate([0,270,90])
rotate([rotate_x,rotate_y,rotate_z])
linear_extrude(height=height1)
text(text1[a],size=size1,font = Font1, halign="center", valign="bottom",spacing = 1,direction="ltr",$fn=100);
}}
module CircleText2() {
for(b=[0:length2]) {
rotate([0,length2/2*rotation2-(b*spacing2),270])
translate([diameter2,0,0])
rotate([0,270,90])
rotate([0,180,180])
rotate([rotate_x2,rotate_y2,rotate_z2])
linear_extrude(height = text_height2)
text(text2[b],font=Font2,size=size2, halign="center", valign="bottom",spacing = 1,$fn=100);
}}
color("white"){
rotate([0,90,0])
CircleText1();
rotate([0,270,0])
CircleText2();
}
linear_extrude(1)
circle(25,$fn=100);
It's pretty easy to make adjustments for the text to fit on any round object from flat to cone shaped to cylindrical:
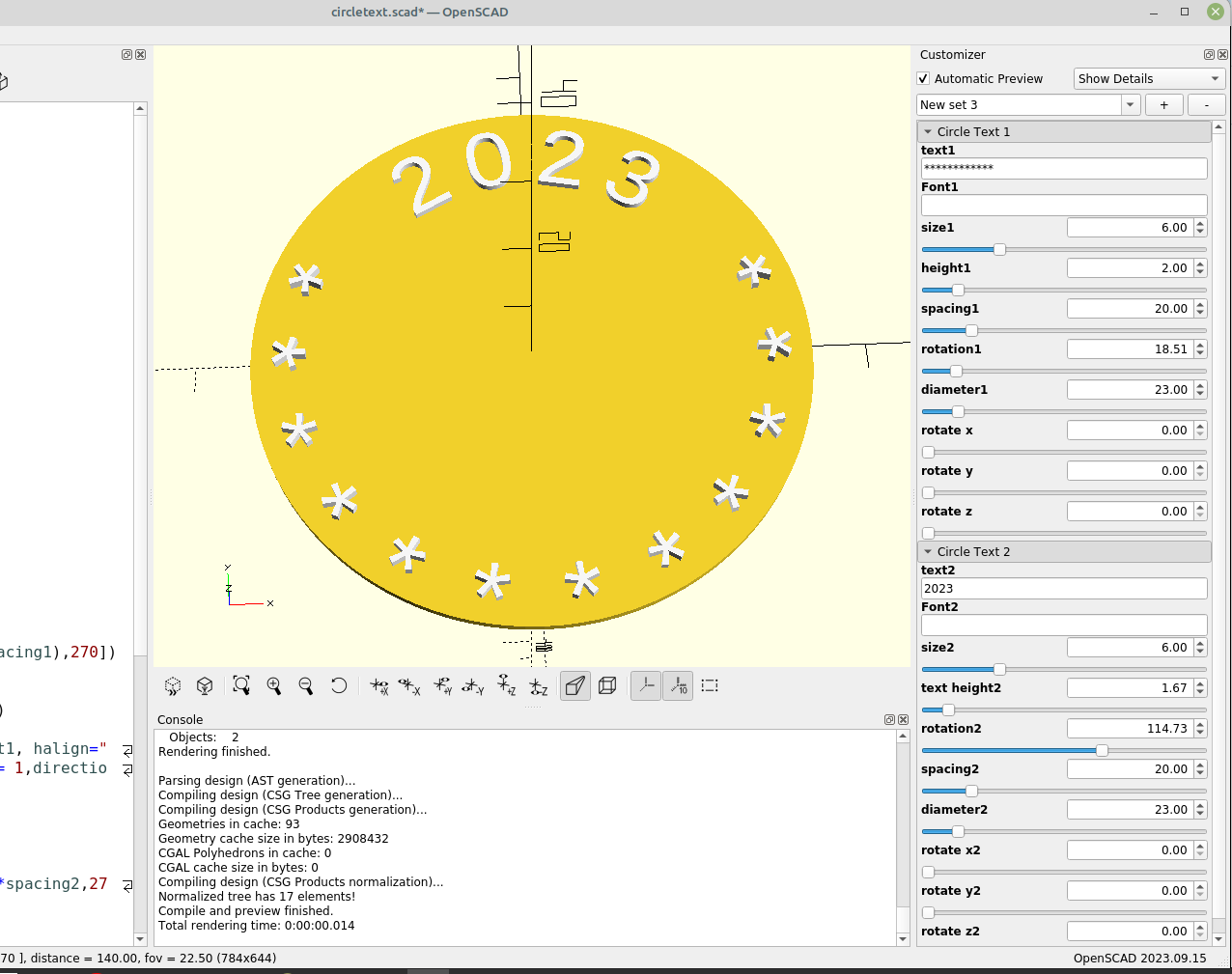
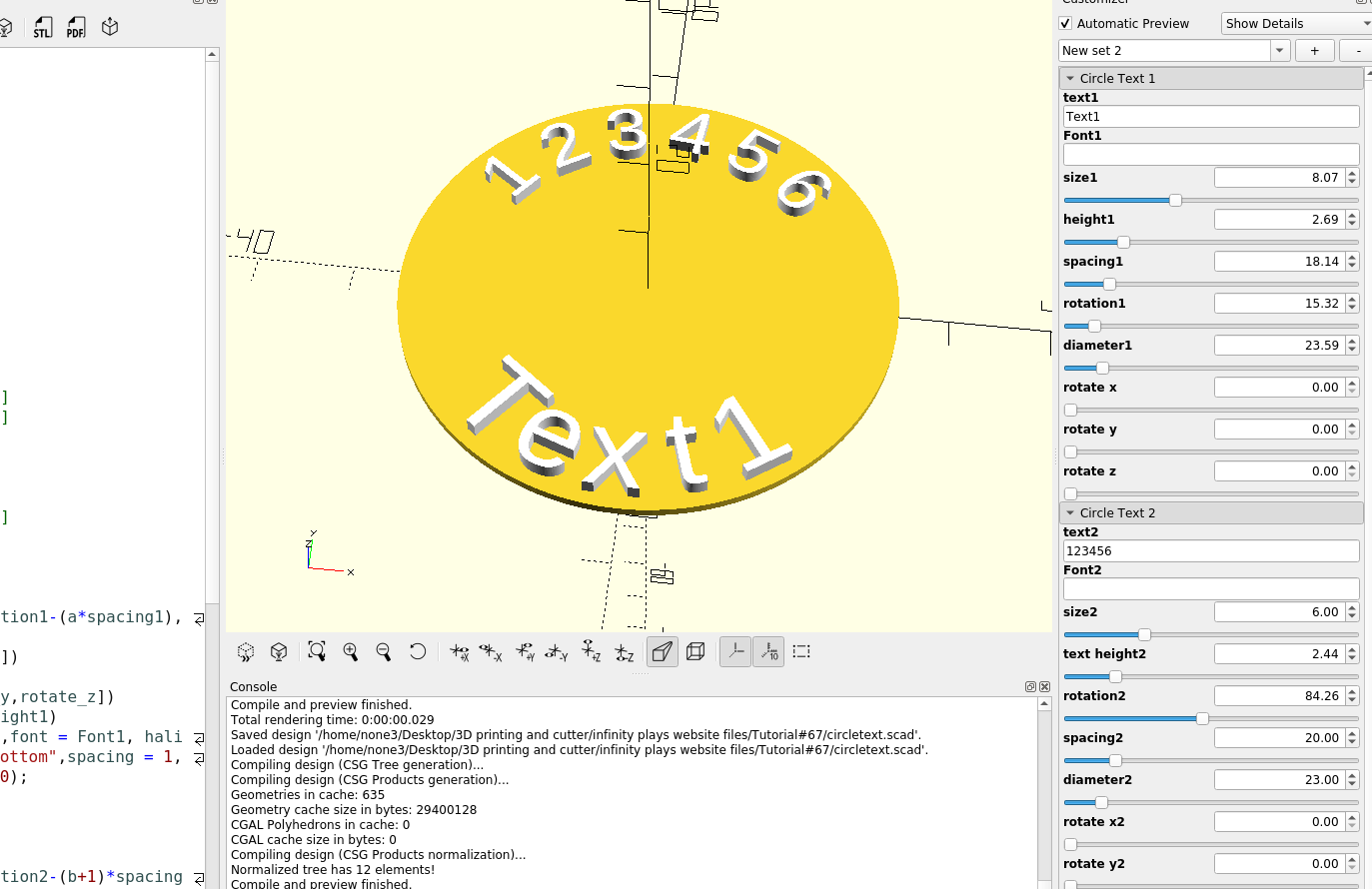
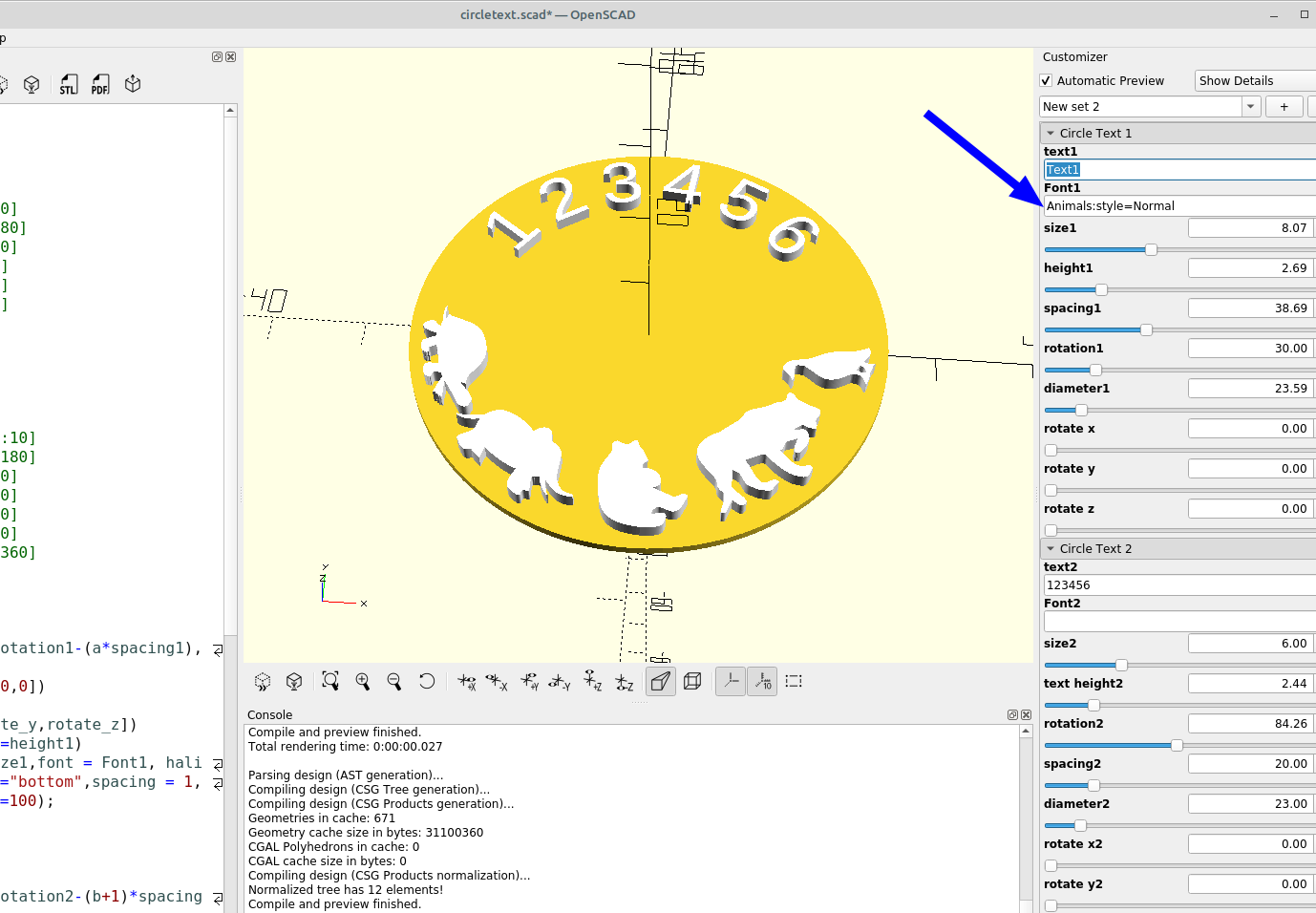
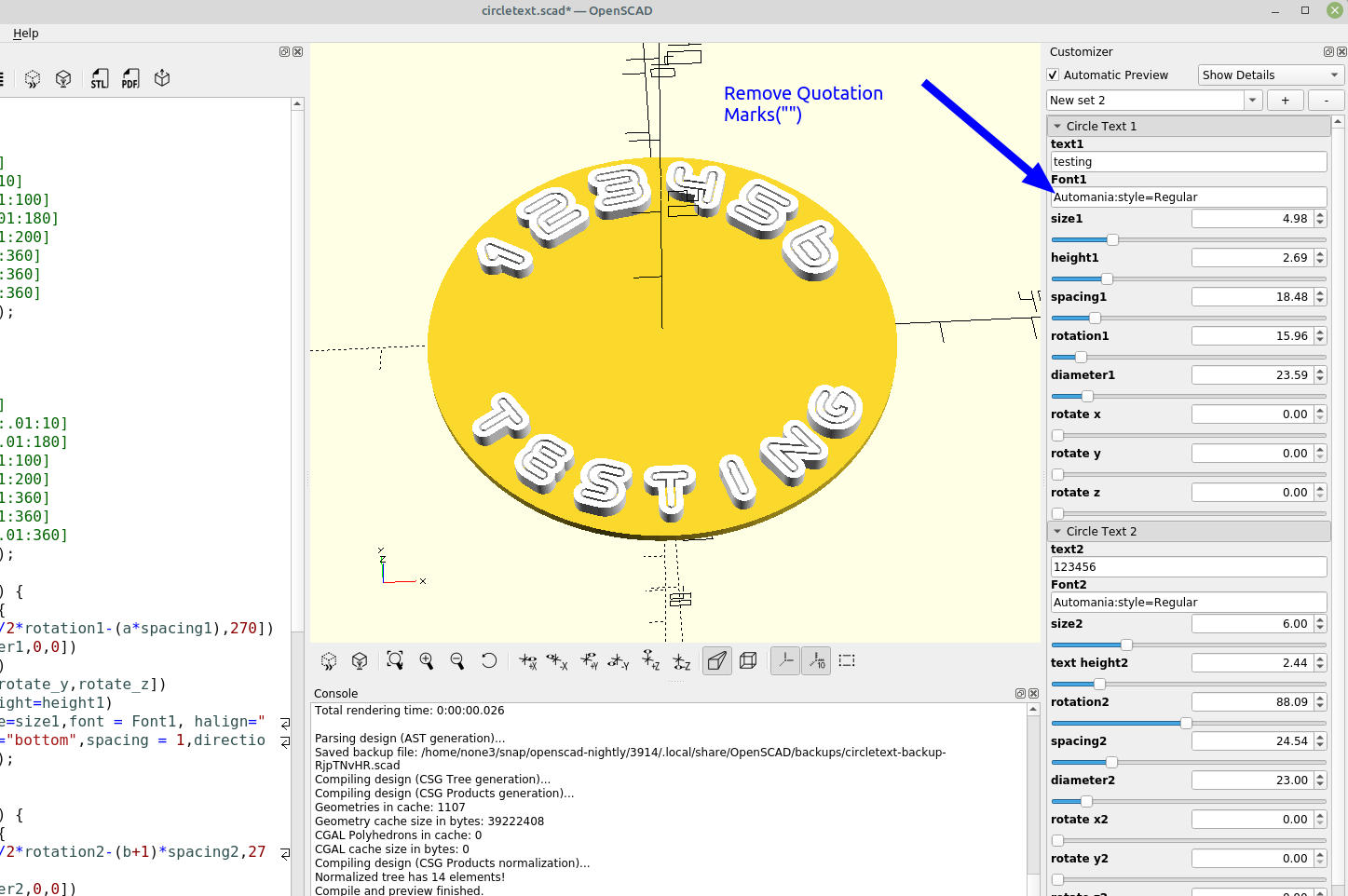
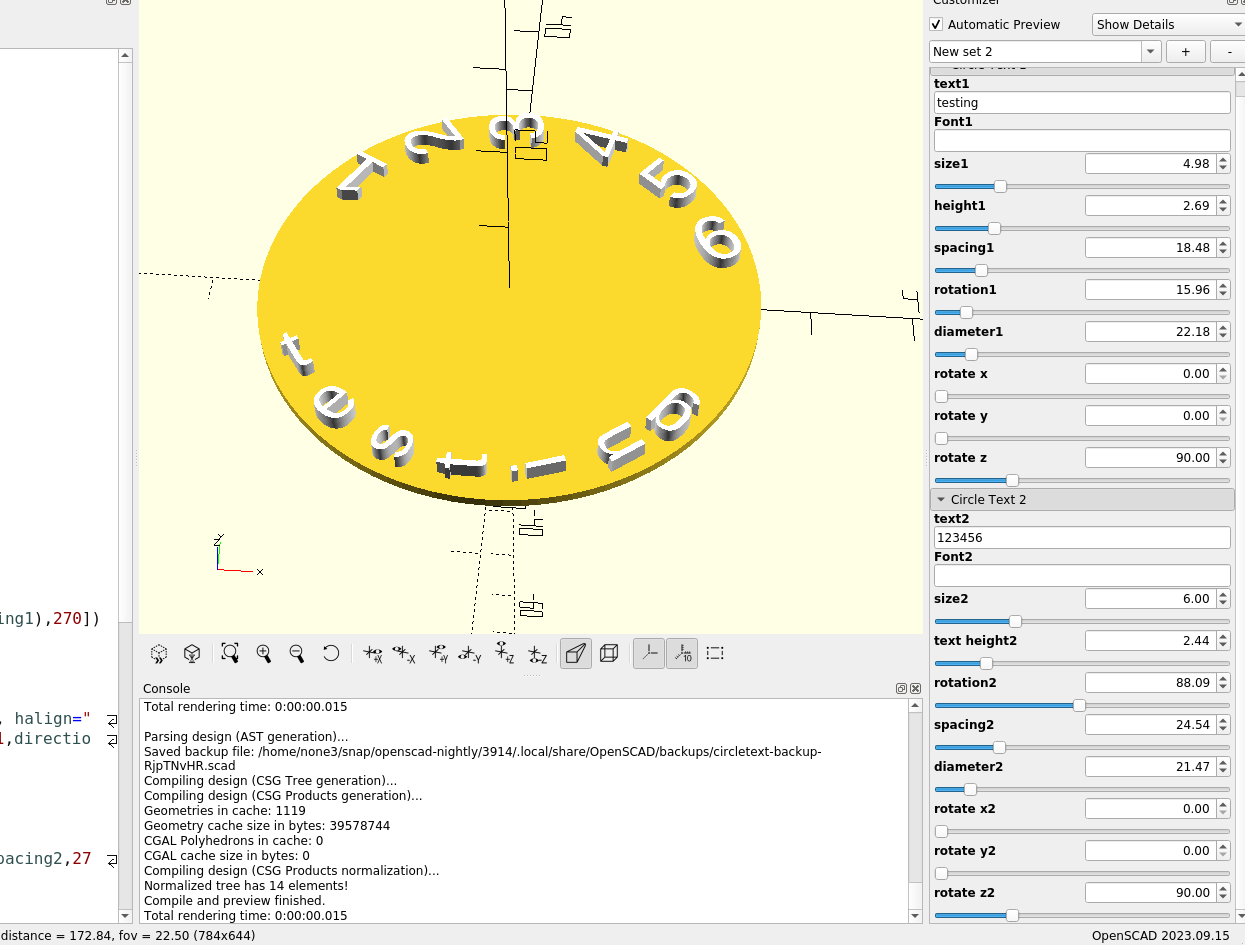
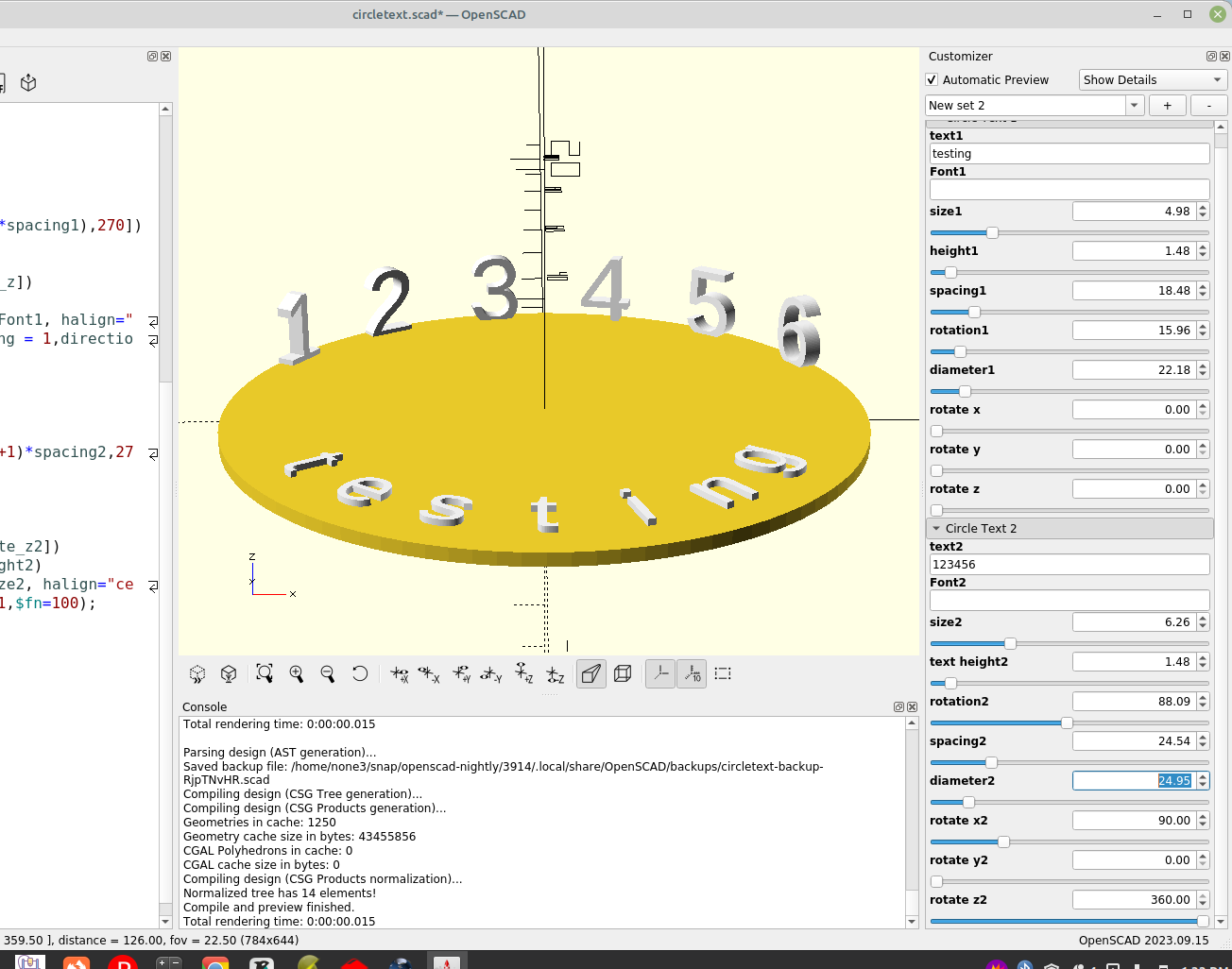