A few days ago I saw a post by krysus on reddit that showed a really neat way to automatically center an stl:
Any protip on centering an imported STL in openscad?
by u/rebuyer10110 in openscad
That post is an excellent example of why I like to use OpenSCAD, if you have the skills and a little imagination you can add your own tools. I wanted to be able to move the stl anywhere I needed to so I can line files up with one another but still move the file to center automatically. The method I use here is similar but a little bit different.
First step is to set the move sliders to zero so when the file is imported the coordinates are the original coordinates:
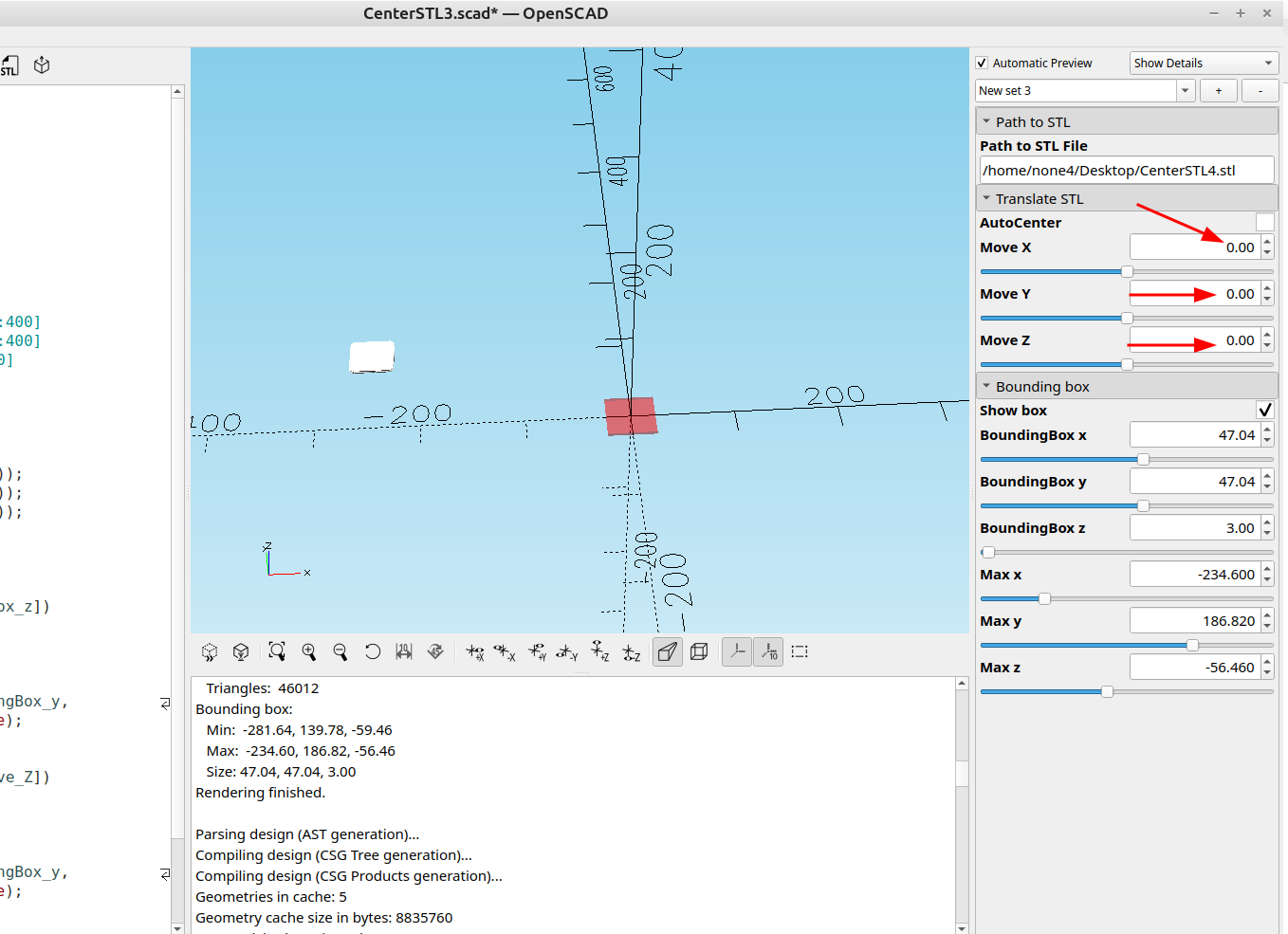
Click on the render button and copy and paste the "Bounding box size" and "max" x, y and z coordinates:
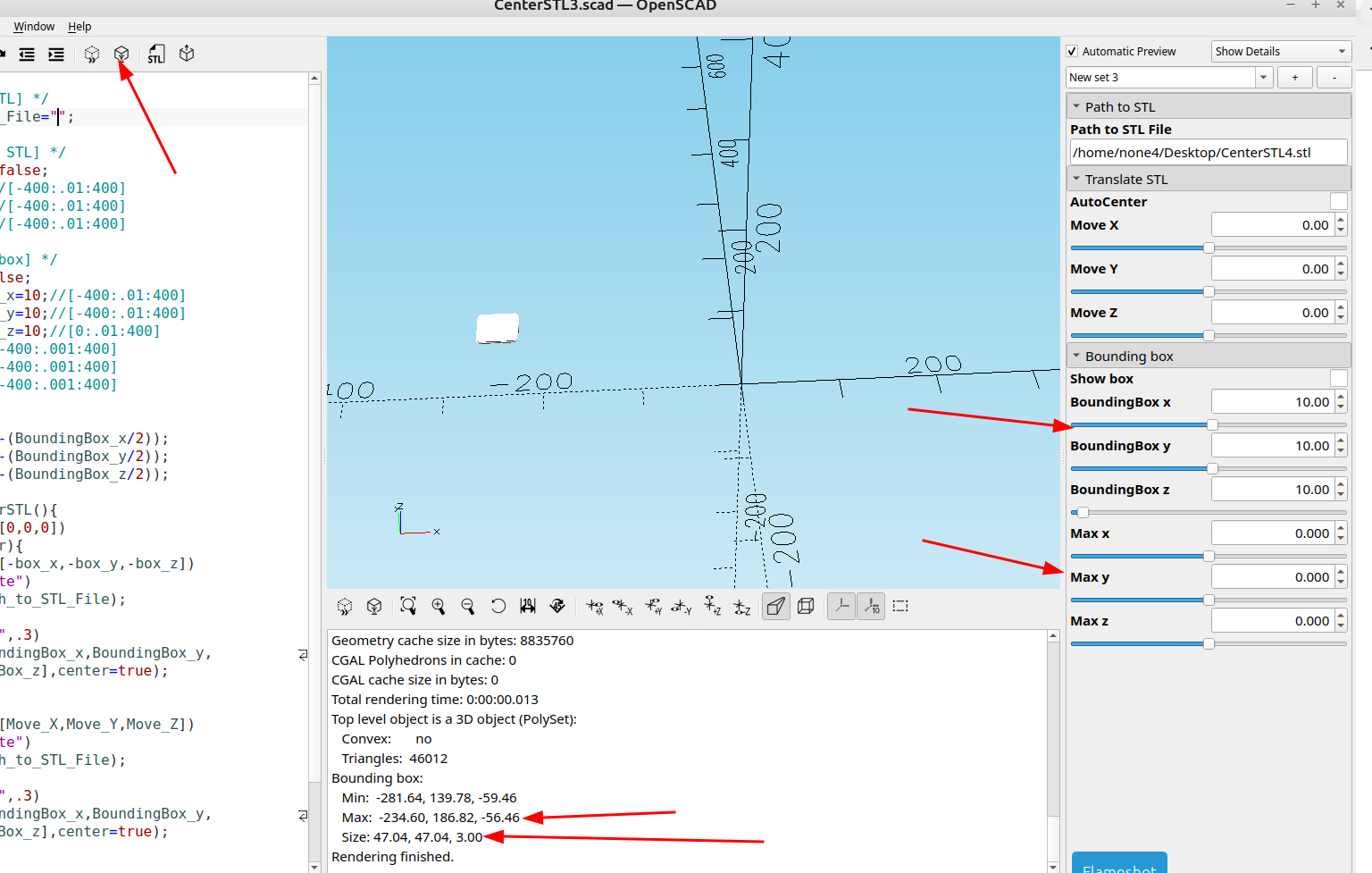
Once they are entered just click the auto center box:
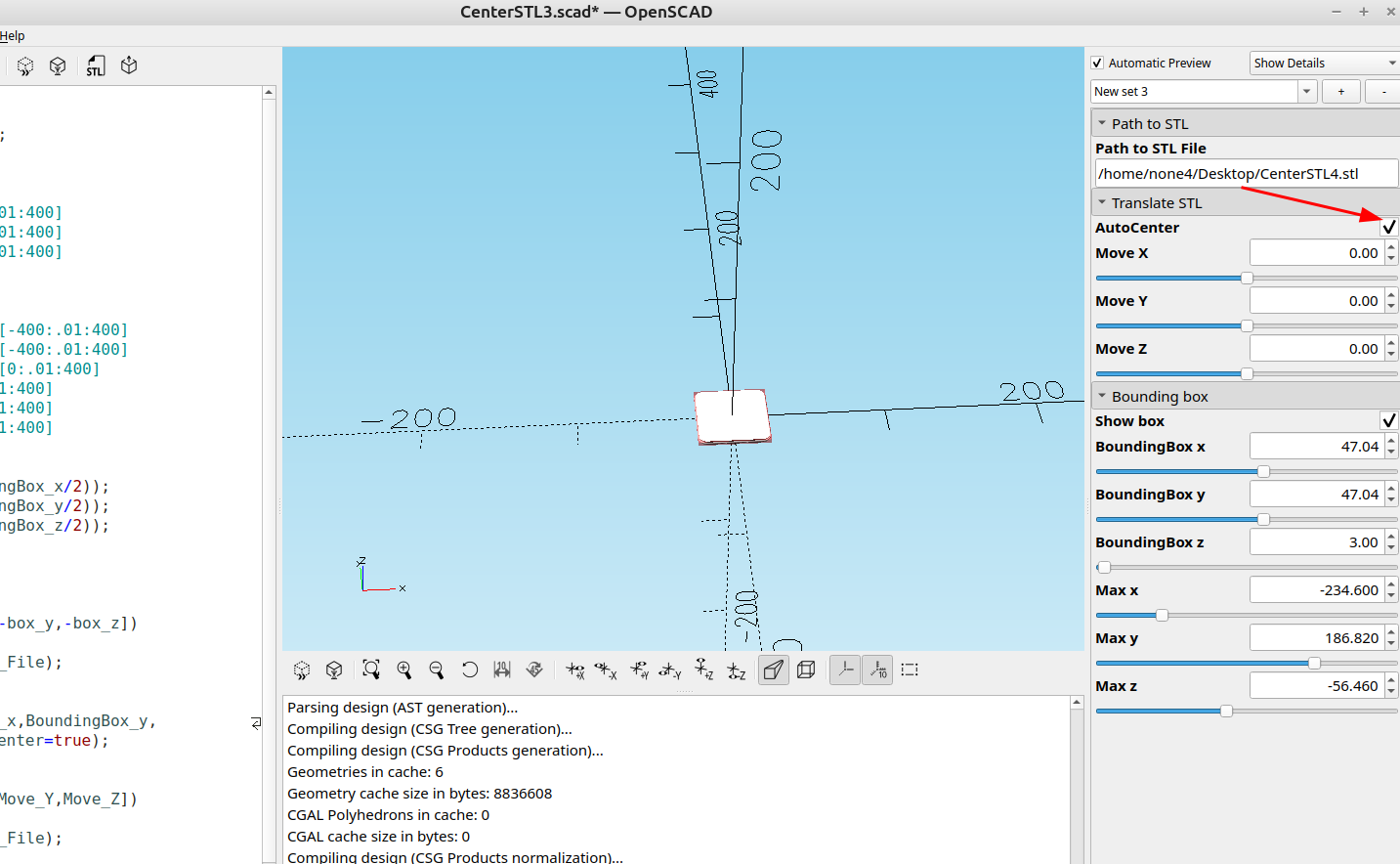
here is the code:
//
/*[Path to STL] */
Path_to_STL_File="";
/*[Translate STL] */
AutoCenter=false;
Move_X= 0;//[-400:.01:400]
Move_Y= 0;//[-400:.01:400]
Move_Z= 0;//[-400:.01:400]
/*[Bounding box] */
Show_box=false;
BoundingBox_x=10;//[-400:.01:400]
BoundingBox_y=10;//[-400:.01:400]
BoundingBox_z=10;//[0:.01:400]
Max_x=0;//[-400:.001:400]
Max_y=0;//[-400:.001:400]
Max_z=0;//[-400:.001:400]
/*[Hidden]*/
box_x=(Max_x-(BoundingBox_x/2));
box_y=(Max_y-(BoundingBox_y/2));
box_z=(Max_z-(BoundingBox_z/2));
module CenterSTL(){
translate([0,0,0])
if(AutoCenter){
translate([-box_x,-box_y,-box_z])
color("white")
import(Path_to_STL_File);
if(Show_box)
color("red",.3)
%cube([BoundingBox_x,BoundingBox_y, BoundingBox_z],center=true);
}
else{
translate([Move_X,Move_Y,Move_Z])
color("white")
import(Path_to_STL_File);
if(Show_box)
color("red",.3)
%cube([BoundingBox_x,BoundingBox_y, BoundingBox_z],center=true);
}}
CenterSTL();
I'm sure there is a way to make this even easier, more experimentation will need to be done.