Quite some time ago I was making a project box customizer but never got around to finishing it, I still need to add standoffs, holes in the lid, etc. But there are a ton of really good OpenSCAD examples available to make project boxes so this one may never get finished.
One thing that might be handy for someone that is trying to figure out how to round the corners for flat plates or boxes is the code that can accomplish this so here is an example:
//
/*[Parts Box] */
Show_parts_box=true;
/*[Box parameters]*/
depth=20;//[1:.01:100]
corner_radius=5;//[0:.01:24]
Wall_thickness=2;//[1:.01:10]
Outer_size_x=50;//[10:.01:200]
Outer_size_y=50;//[10:.01:200]
/*[Lip parameters]*/
lip_depth=1;//[0:.01:10]
lip_width=1;//[0:.01:20]
/*[Lid parameters]*/
Show_lid=true;
Lid_to_printbed=true;
Scale_factor=.99;//[.95:.001:1]
opacity=.5;//[.3:.01:1]
/*[Hidden]*/
$fa=.1;
$fs=0.25;
wall_thickness=[Wall_thickness*2,Wall_thickness*2];
outer_size=[Outer_size_x,Outer_size_y];
module Parts_box(){
if(Show_parts_box)
difference(){
translate([0,0,-depth]){
linear_extrude(height=depth){
offset(r=corner_radius){
square(outer_size-[corner_radius,corner_radius]* 2.0,center = true);
}}}
translate([0, 0,Wall_thickness]){
mirror([0,0,1]){
linear_extrude(height=depth){
offset(r=corner_radius){
square(outer_size-wall_thickness-[corner_radius+lip_width,corner_radius+lip_width]*2.0,center = true);
}}}}}}
difference(){
translate([0,0,depth])
Parts_box();
translate([0,0,depth-lip_depth]){
linear_extrude(20)
offset(r=corner_radius){
square(outer_size-wall_thickness-[corner_radius,corner_radius]*2.0,center = true);
}}}
module Lid(){
if(Show_lid)
color("grey",opacity)
translate([0,0,depth]){
mirror([0,0,1]){
linear_extrude(height=lip_depth){
offset(r=corner_radius){
square(outer_size-wall_thickness-[corner_radius,corner_radius]* 2.0,center = true);
}}}}}
if(Lid_to_printbed){
translate([0,Outer_size_y,-depth+lip_depth])
scale([Scale_factor,Scale_factor,1])
Lid();
}
else{
scale([Scale_factor,Scale_factor,1])
Lid();
}
You can see that by using offset() you can adjust the corner radius on the fly:

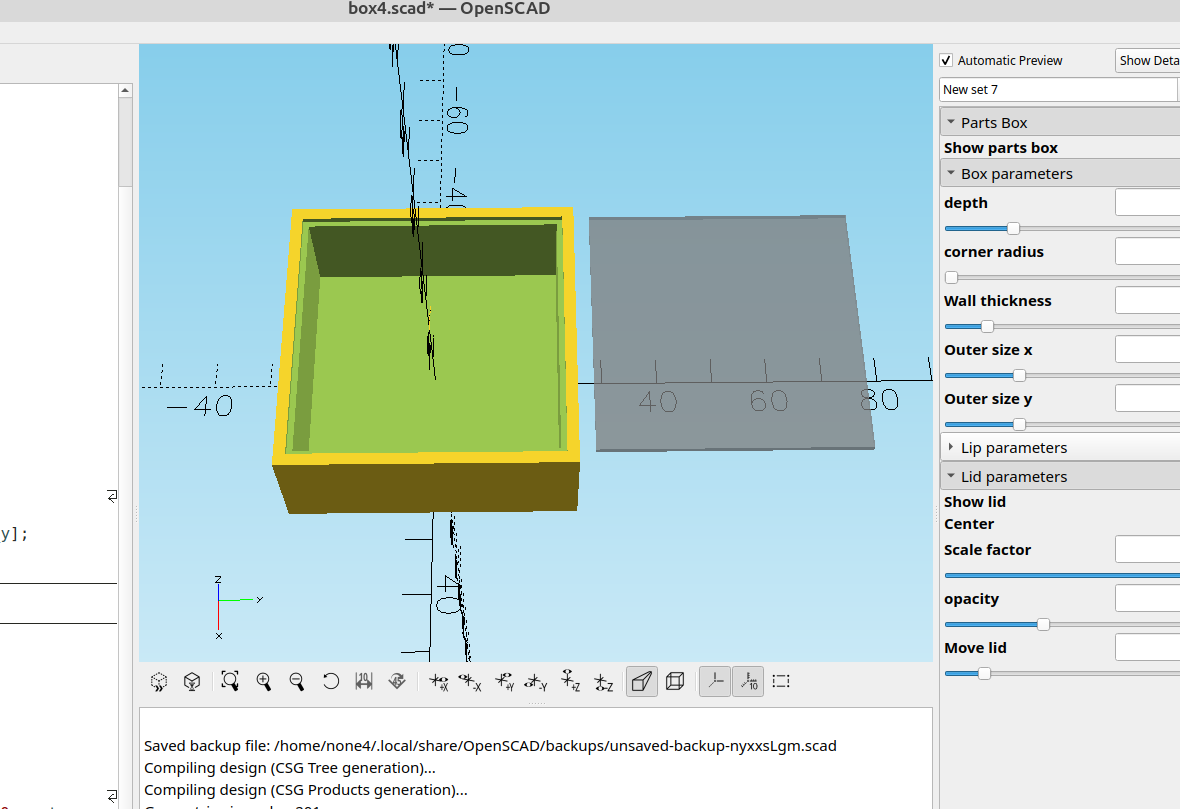
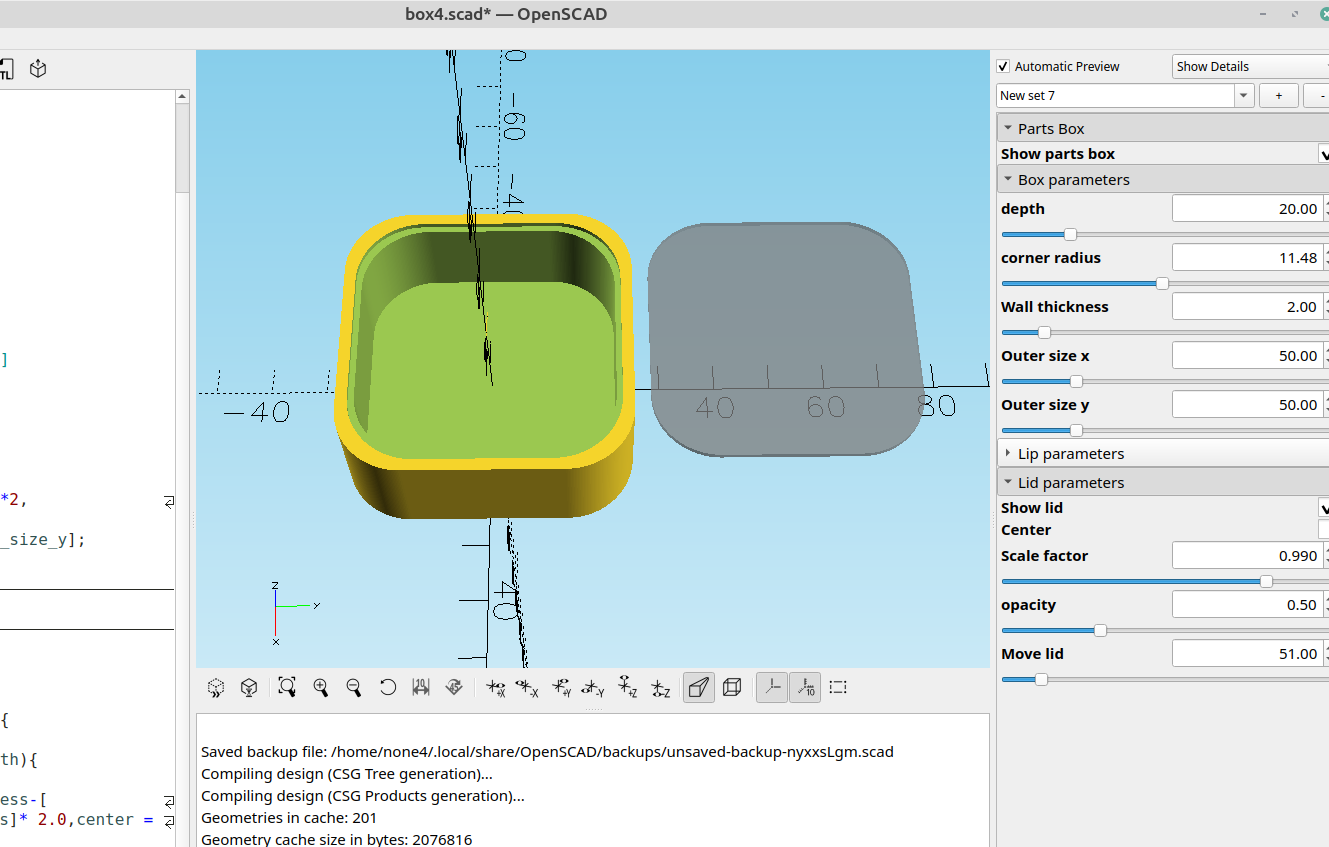
This also makes it easy to match a corner on an existing stl file:
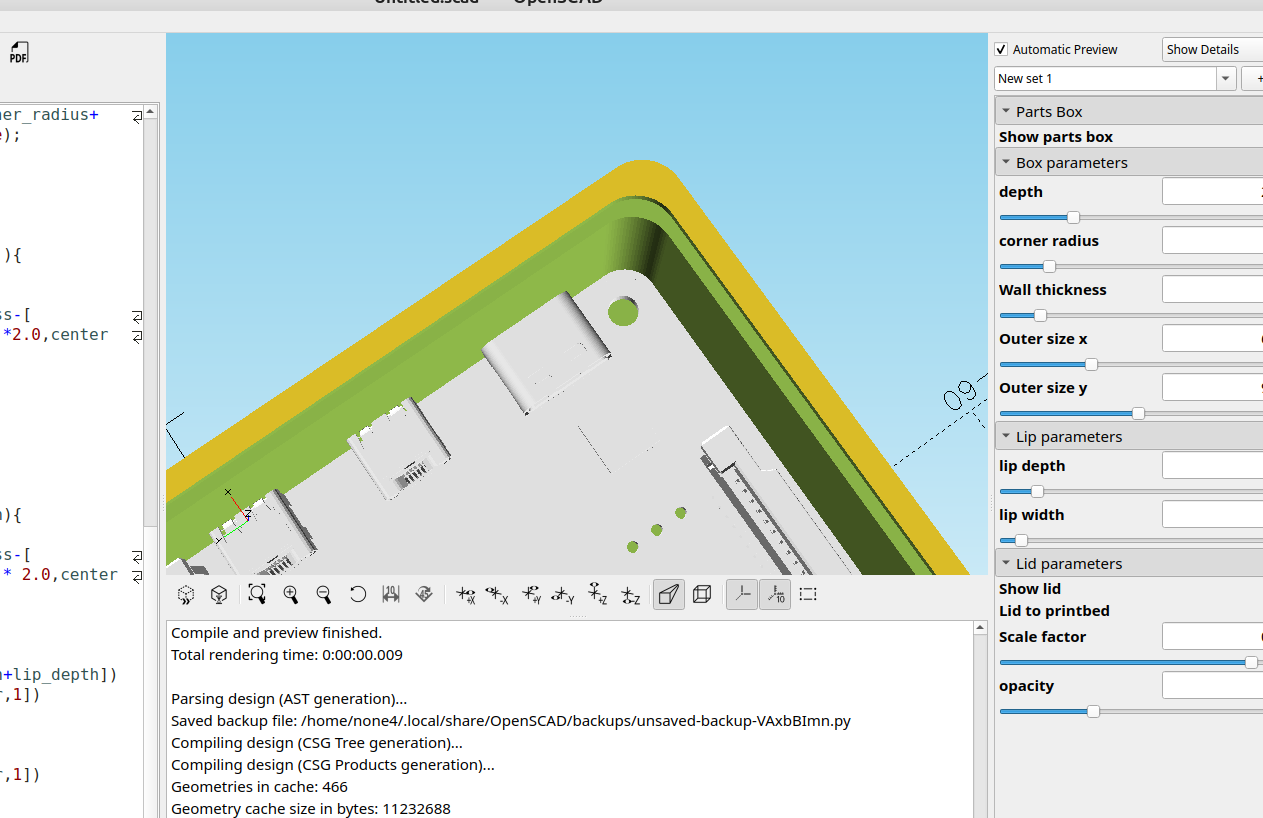
Anyway if you are needing to round the corners of a flat plate or square this is an easy way to do it.